1435
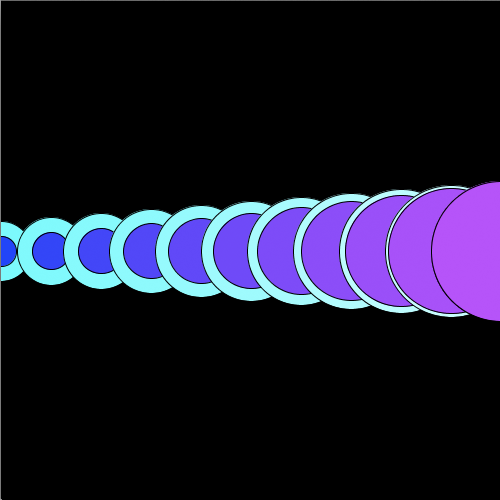
the first half an hour with quil
(ns seni.sketch-1435
#+clj
(:require [quil.core :as q]
[quil.middleware :as qm]
[seni.math :as math])
#+cljs
(:require [quil.core :as q :include-macros true]
[quil.middleware :as qm]
[seni.math :as math]))
(defn setup []
(q/color-mode :rgb)
{:color 0
:angle 0})
(defn draw [state]
(q/background 0)
(q/fill (:color state) 255 255)
(let [canvas-width 500
map-step 10
pos-mapper (math/remap-fn :from [0 100] :to [0 canvas-width])
col-mapper (math/remap-fn :from [0 100] :to [50 200])
inner-mapper (math/remap-fn :from [0 100] :to [0.5 1.0])
radius-mapper (math/remap-fn :from [0 100] :to [30 70])]
(doseq [a (range 0 (+ 100 map-step) map-step)]
(let [radius (radius-mapper a)
inner-radius (* (inner-mapper a) radius)]
(q/with-translation [(pos-mapper a)
(/ canvas-width 2)]
(q/fill (col-mapper a) 255 255)
(q/ellipse 0 0 (* 2 radius) (* 2 radius))
(q/fill (col-mapper a) 0 255)
(q/ellipse 0 0 (* 2 inner-radius) (* 2 inner-radius)))))))
(q/defsketch sketch-1435
:title "Back to basic remapping"
:size [500 500]
:setup setup
:draw draw
:middleware [qm/fun-mode])